Overview of the HTTP pipeline
DjaoDjin helps your Software-as-a-Service (SaaS) product deliver value sooner and more reliably with a Docker container hosting solution ( See djaodjin.com API reference) bundled together with a user-session firewall (djaoapp) that delivers the two main administrative workflows in a SaaS product:
- Subscription-based Access Control
(Pricing, checkout, etc.)
- Role-based Access Control
(Managers, contributors, invites, etc.)
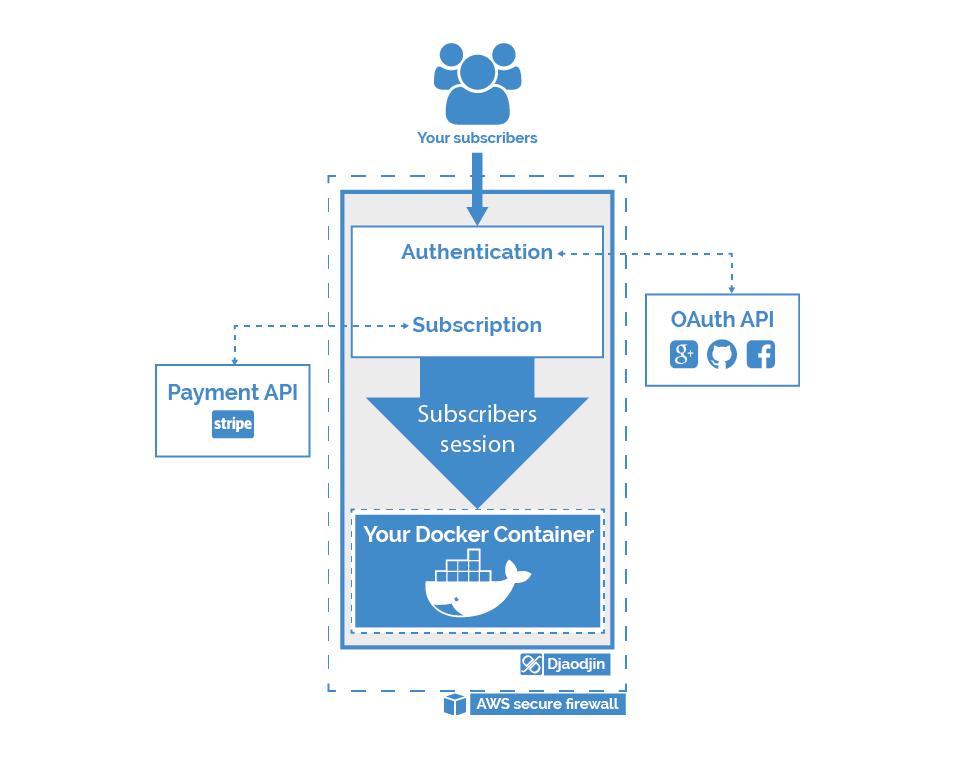
Djaoapp extends your server backend code so all the following HTML pages and API end points are already available to your User experience (UX) designers and front-end developers:
- Authentication
- Accounts management
- Billing
There are no third-party keys to manage in the mobile or web front-end, there are no esoteric CORS configuration to setup... From your User Interface (UI) team, all calls to the djaoapp API looks exactly like a call to your business logic API.
Let's see a high-level view of the HTTP request pipeline and why the microservice approach is the way to build modern SaaS products, then dive into how to integrate djaoapp with your business logic backend.
The HTTP request pipeline ...
We won't talk here about how public static assets (media, css, js files) make it to a user browser through caches, Content Delivery Networks (CDN), nginx reverse proxies, etc. It is a fascinating subject but here we are concerned with dynamic content that often rely on authentication and permissions to access information ultimately stored in a database.
Do It Yourself
If you are writing code in Django, you could integrate the various apps that make up djaoapp directly into a monolithic Web application since all of djaoapp code base is open source. You would though loose on what makes a microservice approach where your business logic and the SaaS administrative logic are their own distinct process:
- Security managed in one place
- Regulatory compliance (GDPR et al.)
- Separate development teams with their own focus
Let's assume you have a SaaS product that helps users manage their todo list.
A user, named Alice, has bookmarked the URL to her todo dashboard
(ex: https://todo.djaopp.com/app/xia/dashboard/
).
She clicks on the bookmark.
The browser issues a DNS lookup to retrieve the IP address of the server
responsible to respond to requests for the domain todo.djaopp.com
(How to use my own domain?),
then sends an HTTP request to that server, asking for an URL path
called /app/alice/dashboard/
.
When the todo.djaopp.com
server receives the HTTP request,
either the user is authenticated or not. In case Alice is not yet authenticated,
she is redirected to the login page.
Djaoapp immediately adds to your application 3 ways for users to authenticate with your SaaS product and all the appropriate themed pages for users to manage their credentials.
- Session Cookies
- JSON Web Tokens
- API Keys
Let's assume you decided to authenticate a user named Alice through
a POST request to /api/auth/
. At this point, your backend
business logic has nothing to do. Everything is handled by djaoapp and
a valid token is returned to the browser.
Now your front-end user interface (UI) is doing a GET request to the backend
server passing the authentication token along, to retrieve the user profile
information (ex. GET /api/users/alice
).
Again, your backend business logic has nothing to do. Djaoapp checks that
the authenticated user (i.e. alice) has permissions to access
the profile information and returns it.
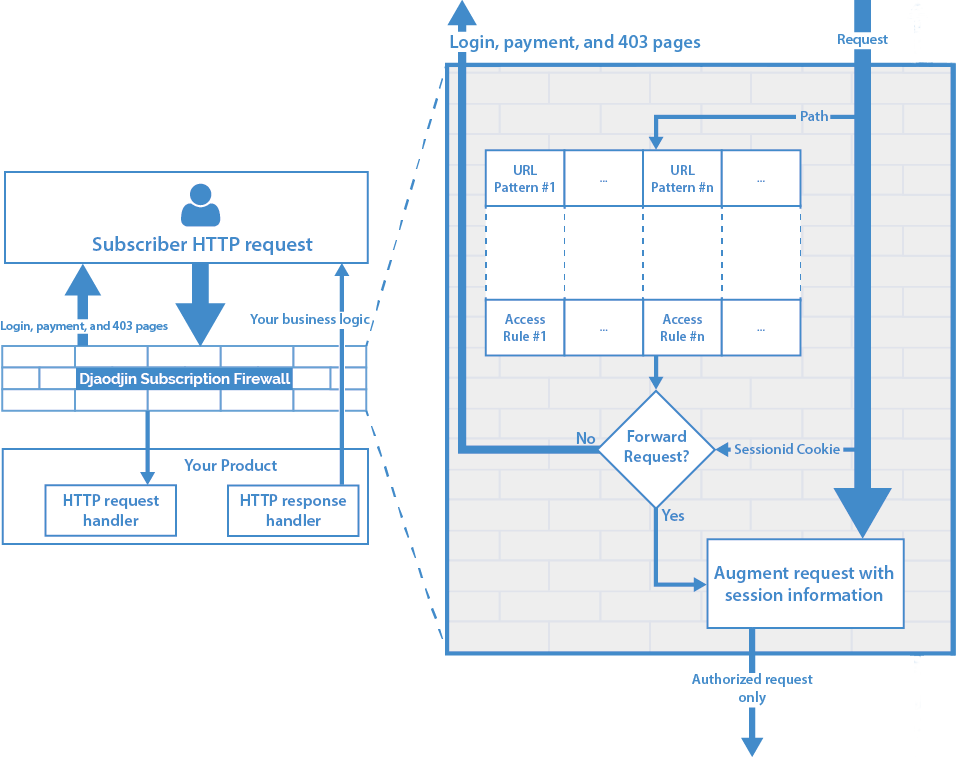
Xia is now authenticated, maybe after a detour through a reset password email loop or a two-factor authentication (2FA) workflow involving a mobile phone.
Your front-end user UI is now doing a GET request to the backend server
to retrieve the todo list for Alice (ex: GET /api/todos/alice/
).
This is an actual call to the value proposition of your SaaS product.
The HTTP request flows through djaoapp which:
In Today's environment, controlling access to a resource is not straightforward. There are many historical artifacts on how the Internet works with potential for a bad actor to impersonate Xia with techniques such as cross-site request forgery (CSRF), cross-site scripting (XSS), or a bad actor to prevent access to a service from legitimate users through techniques such as Denial of Service (DoS).
- checks the authenticated user has permissions to make that request (see defining access rules).
- retrieves personal information from djaoapp database about the authenticated user (full name, roles, etc.)
- encodes the authenticated user personal information into either a session cookie or a JSON Web Token
- forwards the original HTTP request, modified to include the previously generated session data as HTTP Headers. (there is a Testing section under the Rules dashboard that will generate headers passed to your backend.)
Once your backend server receives the djaoapp forwarded request, it will decode the session data with its DjaoDjin secret key (see Frameworks integration) and process the request according to your business logic.
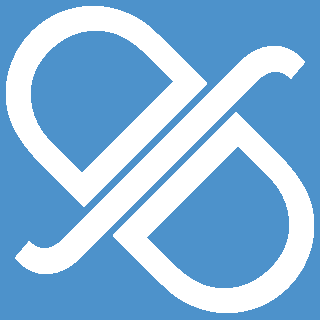
GET /api/todos/alice/ HTTP/1.1
Authorization: Bearer <JWT>
Code inside djaoapp is triggered that decodes <JWT> to
{
"username": "alice"
}
After djaoapp applies the access rules (defined for your backend logic in the Rules dashboard), it decides to forwards the request as:
GET /api/todos/alice/ HTTP/1.1
Authorization: Bearer <JWTBackend>
Where <JWTBackend> can be verified with your DjaoDjin secret key and the session data decodes to:
{
"username": "alice",
"roles": {
"manager": [{
"slug": "alice",
"printable_name": "Alice Lee",
"subscriptions": [{
"plan": "open-space",
"ends_at": "2020-12-31T00:00:00Z"}]
}]
}
}
The above examples is interpreted as: “A request user alice is a manager of the billing profile alice, itself subscribed to the open-space plan.”
A support team member should be able to access a customer profile and billing information but a customer should not be able to access another customer profile - obviously. When you have a single integrated database, there is a non-negligeable risk, especially as business rules complexify, that through bugs and/or SQL injection, security and privacy of customer data are not maintained. By separating the concerns between djaoapp and your application, security and privacy are easier to enforce.
Frameworks integration
Decode Authorization Header
When you are hosting your business logic as a Docker container on djaodjin.com, all HTTP requests fall through djaoapp first before reaching your application. DjaoApp forwards the original HTTP request, modified to include the user session data encoded as a JSON Web Token (JWT) passed in the Authorization HTTP Header.
You need to decode the JWT in the Authorization Header and verify its validity using your application DjaoApp secret key.
We provide libraries that make it straightforward to integrate the djaoapp session manager in front of many popular frameworks. (Example: Django)